Sends bundle analysis metrics to your InfluxDB database to create custom dashboards, correlate the data with other metrics, or create custom alerts.
Metrics forwarding is available for Open Source, PRO and Enterprise plans.
After every processed job, RelativeCI sends a measurement to InfluxDB that includes all bundle analysis metrics and the tags that identify your project and the job:
Forwarded metrics and tags
Metric | Unit | Description |
---|---|---|
bundleSize | byte | Total bundle size |
initialSizeJS | byte | Total size of the initial JS assets |
initialSizeCSS | byte | Total size of the initial CSS assets |
cacheInvalidation | percentage | Percentage of changed assets |
assetCount | number | Total number of assets |
chunkCount | number | Total number of chunks |
moduleCount | number | Total number of modules |
duplicateModuleCount | number | Total number of duplicate modules |
duplicateCode | percentage | Percentage of duplicate code |
packageCount | number | Total number of packages |
duplicatePackageCount | number | Total number of duplicate packages |
bundleSizeJS | byte | Total size of the JS assets |
bundleSizeCSS | byte | Total size of the CSS assets |
bundleSizeIMG | byte | Total size of the image assets |
bundleSizeMEDIA | byte | Total size of the media assets |
bundleSizeFONT | byte | Total size of the font assets |
bundleSizeHTML | byte | Total size of the HTML asets |
bundleSizeOther | byte | Total size of other assets |
Every measurement is tagged with the following data:
Tag | Description |
---|---|
organization | The repository owner value |
repo | The repository name |
name | The RelativeCI project name |
branch | The job branch name |
How to configure your InfluxDB connection
To configure Metrics Forwarding for InfluxDB, navigate to your project Settings -> Integrations -> Metrics Forwarding - InfluxDB and add your InfluxDB 2.0 database connection details:

Debugging
In case of errors, you can browse the integration logs on Settings -> Integrations -> Metrics Forwarding - InfluxDB -> Logs and preview the JSON response.
Examples
Bundle size single stat

from(bucket: "relative-ci-data") |> range(start: v.timeRangeStart, stop: v.timeRangeStop) // Filter by measurement |> filter(fn: (r) => r["_measurement"] == "relative-ci") // Filter by metric name |> filter(fn: (r) => r["_field"] == "bundleSize") // Filter by RelativeCI project name |> filter(fn: (r) => r["name"] == "web-app") // Filter by RelativeCI job branch |> filter(fn: (r) => r["branch"] == "master")
// Select the last value |> last()
To display the value using a custom unit, add the bytesToMiB
function and use it to transform the value:
bytesToMiB = (tables=<-) => tables |> map(fn:(r) => ({ r with _value: string( v: float(v: (r._value * 100 / 1048576)) / 100.0 ) + "MiB" }))
from(bucket: "relative-ci-data") |> range(start: v.timeRangeStart, stop: v.timeRangeStop) // Filter by measurement |> filter(fn: (r) => r["_measurement"] == "relative-ci") // Filter by metric name |> filter(fn: (r) => r["_field"] == "bundleSize") // Filter by RelativeCI project name |> filter(fn: (r) => r["name"] == "web-app") // Filter by RelativeCI job branch |> filter(fn: (r) => r["branch"] == "master")
// Select the last value |> last()
// Format output value |> bytesToMiB()
To allow you to select the project and the job branch, add two corresponding dashboard variables and reference them in the query:
import "influxdata/influxdb/schema"schema.tagValues(bucket: "relative-ci-data", tag: "name")
import "influxdata/influxdb/schema"schema.tagValues(bucket: "relative-ci-data", tag: "branch")
bytesToMiB = (tables=<-) => tables |> map(fn:(r) => ({ r with _value: string( v: float(v: (r._value * 100 / 1048576)) / 100.0 ) + "MiB" }))
from(bucket: "relative-ci-data") |> range(start: v.timeRangeStart, stop: v.timeRangeStop) // Filter by measurement |> filter(fn: (r) => r["_measurement"] == "relative-ci") // Filter by metric name |> filter(fn: (r) => r["_field"] == "bundleSize") // Filter by RelativeCI project name dashboard variable |> filter(fn: (r) => r["name"] == v.relativeCIProjectName) // Filter by RelativeCI job branch dashboard variable |> filter(fn: (r) => r["branch"] == v.relativeCIJobBranch)
// Select the last value |> last()
// Format output value |> bytesToMiB()
Initial JS size values over time
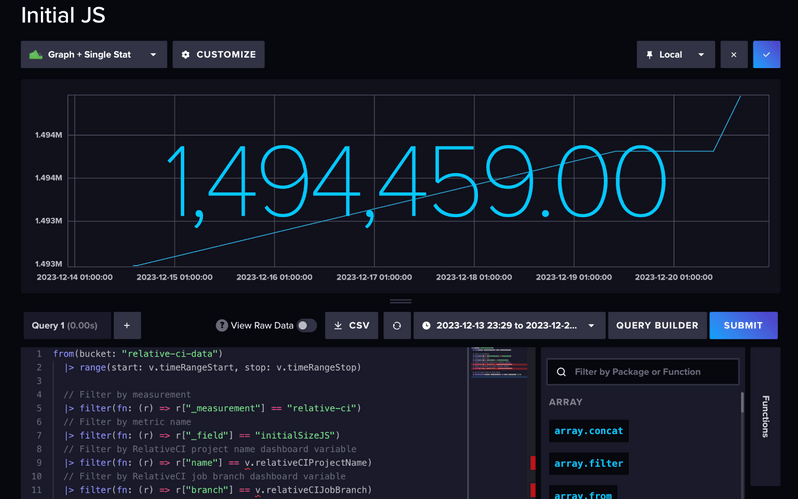
from(bucket: "relative-ci-data") |> range(start: v.timeRangeStart, stop: v.timeRangeStop)
// Filter by measurement |> filter(fn: (r) => r["_measurement"] == "relative-ci") // Filter by metric name |> filter(fn: (r) => r["_field"] == "initialSizeJS") // Filter by RelativeCI project name dashboard variable |> filter(fn: (r) => r["name"] == v.relativeCIProjectName) // Filter by RelativeCI job branch dashboard variable |> filter(fn: (r) => r["branch"] == v.relativeCIJobBranch)
// Get the latest value for every period |> aggregateWindow(every: v.windowPeriod, fn: last, createEmpty: false)